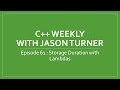
Another way that one might create a stateful lambda in C++11 by manipulating the storage duration of the variables in the lambda.
Another way that one might create a stateful lambda in C++11 by manipulating the storage duration of the variables in the lambda.
I continue my series on embedded C++ with an example of how we can merge register accesses and save a few more bytes.
I continue my series on embedded C++ with an example of how templates and constexpr can provide flexibility while reducing the compile size and increasing performance.
In this episode we get set up with using the latest C++ features to program a chip which has only 2KiB of flash and 128Bytes of RAM available.
In this episode I break down how some C++ features are defined in terms of goto, so we are essentially using goto throughout our code.
In this episode we play with llvm-cbe, the C back-end for LLVM, which allows us to essentially compile C++ code into C code.
In this episode we try to see just how far we can take the idea of a “stateful lambda” by building one that can be manipulated and interacted with from the caller side.
Now that we know why we would inherit lambdas, and merge them into one implementation (to make a visitor, for instance), how and why does this compare to using a generic lambda? What advantage does one have over the other?
In this episode I build from episode 40’s discussion on inheriting lambdas and show how several C++17 features (including variadic using
declarations) can be combined to create a succinct merging of lambdas.
Last episode I showed you why you should be taking Visual C++ seriously. This episode I show you my personal pet peeve with Visual Studio that has caused many of my portability issues with cross platform development.
In this episode I demonstrate why you should take Visual C++ seriously and make it a part of your automated build environment.
In this episode I demonstrate how to build a random maze generator (and solver) that can be executed at compile time with constexpr
.
In this episode I demonstrate a constexpr enabled random number generator. I then it to generate a different random number sequence each time it is compiled.
In this episode I demonstrate how C++17’s support for lambdas in a constexpr context can clean up many constexpr use cases.
In this episode I discuss how (and why) you can inherit from a lambda function along with possible use cases.
C++17’s automatic class template deduction feature brings with it a new sub-feature called “deduction guides” which are used to help the compiler with class template type deduction. I give examples of what they are and how they are used in this episode.
Experimenting with C++17’s class template argument type deduction feature and learning what its limitations are.
Part 3 of our basic introduction to understanding the assembly output from your compiler. In this episode we do some reverse engineering of other non-Intel architectures.
Review of using Visual C++ 1.52 from 1993. This is the oldest version of Visual C++ available on MSDN.
A comment was made on my YouTube channel that destructuring in C++17 would be much more useful if it worked with standard containers. At the moment I responsed that this would be someone impossible to get right without adding too much overhead.
C++17 has not only added many new interesting things, it has removed and deprecated a few outdated features as well.
In this SE we follow up episode 18’s constexpr if with some concrete examples of where it can be used in existing code.
Last week we discussed how to use std::bind
. this week we discuss why you should never use std::bind
Many of the different ways you might implement a Fibonacci sequence in C++ plus one you’ve probably never considered.
In this episode I correct some of the details of the “std::future Quick Start” episode while expanding on the info provided.
It is possible to perform some form of the variadic folds that are coming in C++17 with the C++11 and C++14 compilers that we have available today by using just a little bit of creativity.
In this episode Jason wraps up what we’ve learned so far about variadic templates and shows some additional techniques to consider.
In this episode Jason gives a very fast quick-start to what std::future is and how to use std::async to run a function in another thread.
In this episode Jason discusses some of what C++ name mangling is, and how to demangle names at runtime.
In this episode I give a brief introduction to C++ variadic templates and cover some compile time and runtime performance considerations.
Note: this article was originally written in 2005. I’m importing some missed content into my new site and this is one of those articles. Much of the information in this article is out of date, but it still has some relevance. To see my personal take on the best way to embed scripting in C++, checkout ChaiScript.
Back in 2008 I wrote an article on template code bloat. In that article I concluded that the use of templates does not necessarily cause your binary code to bloat and may actually result in smaller code! This ended up becoming one of my more significant articles and has been referenced on wikipedia.
IIFE (Immediately-Invoked Function Expression) is a common tool used in JavaScript. The idea is to both define an anonymous function and call it in the same expression. The point is to produce a local scope for variables so they do not pollute the global scope.
If you make use of inheritance, it’s likely that you need to provide a virtual destructor for your classes. Modern compilers will warn if you do not, but this is a pretty recent development.
July 13, 2009 Reddit covered Release 1.0 of ChaiScript. Many things changed in the last 5 years. Features added, dependencies removed, and performance increased. With all of the changes, we decided it was time to provide a 5 year retrospective and give the world a second first-look at ChaiScript.
We’ve already covered C++11 decltype and why you should start using C++11 features today. Now we will move on to one of the most widely supported C++11 features: the new auto
keyword.; auto
is supported currently by g++ as of 4.4, clang and msvc since 10. So you are safe using it today if you don’t need to support any older compilers. The auto
keyword is used to let the compiler determine the type of a variable. This seemingly simple concept can really help clean up your code in some situations. For example:
Recently, while watching the GoingNative conference, I learned about the new std::shared_ptr
helper function std::make_shared
.
Quick, which language is the following code written in?
Every major platform and compiler now supports some aspect of the new C++ standard accepted in 2011. This means it is currently possible to write code that uses some of C++11 while maintaining cross-platform compatibility. Why should you care?
Neither C++ Coding Standards nor Effective C++
addresses the question of which float point type is best to use and in what situations. There are three floating point types in C and C++:
decltype
is a type specifier introduced in C++11. It behaves like a function that evaluates to the type of an object at compile time. This article is helping provide some more background information necessary for the more meatier C++11 articles to come.
In this article we are going to introduce the concept of C++ Template Partial Specialization. This is meant to be just a primer on the topic and not exhaustive. The examples here will be used and referenced in later articles. A series of discussions about C++11, now that the language has been finalized, will be coming shortly. In C++ a template class such as this:
I just spent the better part of the day debugging an insidious little bug. It really shouldn’t have taken that long… I even had unit tests in place that covered the code in question! Right!?
Microsoft recently posted a video on Channel 9 and their blogs describing the upcoming work they have planned for C++. The abstract of the video tries to point out Microsoft’s unfailing devotion to C++ development:
Considering the number of articles and polls we come across asking if C++ is dying or dead combined with the decrease in C++ job posting I have personally noticed, C++ sure is alive and well in the AI frontier. C++ takes or ties the top 27 places at the 2010 Google AI Challenge
In the context of the rest of the Nobody Understands C++ series, I feel like this one is redundant. But it seems like it needs to be said. C++ is not an object oriented programming language. C++ is a multi-paradigm language that supports most of the major programming paradigms that have been widely accepted. Specifically, C++ supports:
As others have pointed out, the Final Committee Draft (FCD) of the next C++ standard is now available for anyone to download.
GCC 4.5.0 has frozen and the release has made it to thefront page. This is significant to me for 2 main reasons.
This past Saturday I attended the Developer Day meeting in Boulder, CO. Overall the day was beneficial and interesting even tho it was more dynamic languages centric and very few things applied directly to my C++ development. I did present ChaiScript during a lightning talk.
I was recently at a talk where the speaker was discussing the history of C++. He argued that one problem with C++ was that its design requirements included backward compatibility with C code, and one fallout of this was the requirement to support all previous types of error handling as well as adding exceptions. That is, C++ supports:
There has been much discussion over the years about the usefulness of operator overloading in C++ as well as the ability to get it right.
I have been working for the past few weeks on ChaiDraw. ChaiDraw is an application that’s both meant as an educational toy and as a showcase for how to effectively use ChaiScript in your application.
Jon and I released the first release of ChaiScript earlier today. ChaiScript is designed to make it trivially easy to use scripting in your C++ application and to expose your C++ to the scripting system.
I often mention books that I use as resources, but rarely websites. It seems, on average, that online C++ resources are lacking. However, there are a few that I have found that provide quite valuable insight.
colorgcc is an awesome little tool for colorizing the output of gcc and g++, making compiler errors and warnings much easier to spot.
If you have dozens or hundreds of threads doing work they are probably producing some sort of output. I’m not referring to logger output, but to permanent storage, perhaps in a database. If you cannot store the data as quickly as you produce it, you will eventually run into problems. If you are just barely able to keep up with data storage then your scalability will be limited. In the system I am working on poor database performance caused a cascade of errors, making the root cause very difficult to track down. The queue used to store database inserts was growing so large that it was causing out of memory errors which resulted in new
s returning null. The library calling new
did not check the return value, which then caused a segmentation fault when the library attempted to use the pointer.
While debugging my massively multithreaded C++ application I would notice times where the application would seem to pause for a few moments. During one of these pauses I halted the application and attached to it with the debugger (GDB). From within GDB I listed (info threads
), switched to (thread
) and looked at the stack (bt
) of each thread running. I saw something surprising and very telling. Nearly every single thread that was supposed to be performing work was actually blocked on a mutex inside of either malloc
or free
.
If your application does not scale as your threads increase, you should check the code to make sure there are no hidden mutexes limiting your concurrency.
Let’s not overcomplicate things here. If you have 160 threads all trying to run concurrently, even if they are doing little to no work, they are all still doing some work. There’s no reason to make the threads work harder than they need to. Before we get too far, let’s make sure that our compiler optimizations are enabled. It’s possible that compiler optimizations can obscure debugging information or introduce runtime errors if you are doing things that are “tricky” in your C++ code. Be careful, at the first sign that the optimizations are causing problems, back them off. As far as I know, all optimizations are generally considered to be safe, but add to compile time and to the size of the compiled code. Hopefully, if you read this blog often, you do not try to be too clever in your code and are sticking to the spec. If you do not specify any compiler options to GCC you are telling the compiler to build for i386 architecture with no optimizations. Modern CPU’s have far more capabilities than the i386 and taking advantage of them is a good idea. A good start is to enable the most common optimizations and tune the compiled code for a modern CPU architecture: ` gcc -O2 -mtune=pentium3`
This is the beginning of short series of articles related to optimizing massively multithreadded C++ applications. I’m not entirely sure what the exact definition of “massively multithreaded” is, but for our purposes, let’s assume at least twice as many threads as CPU cores. Having so many OS level threads may not be the most efficient way of handling concurrency, but it is legitimate if most of your threads spend most of their time waiting. They may be waiting on a timeout, as in a timer thread that fires on regular intervals, or a message processing thread that is waiting on IO. The application that I am currently optimizing and debugging has anywhere from 25 to 165 threads running depending on the current parameters of the system. All of them are waiting on something: timers, network IO or message queue condition variables that signal the arrival of new messages. There were several hurdles to getting this configuration to execute efficiently. For this intro article, I’m going to start with two links that I actually found after I had done most of the optimization, and were not directly useful to me.
Andrei Alexandrescu gave the keynote speech at Boostcon 2009. The speech’s title was “Iterators Must Go.” I did not have the opportunity to attend this year’s Boostcon, but the slides of the keynote are available online.
In C++, the virtual keyword, when applied to class methods, aids in polymorphism. If a method is declared to be virtual, the most derived version of the method is executed when a call is made. If a method is non-virtual, the specific version that is called depends on how the method is called. If the method is called via a pointer to the base class, the base class method is called, if by the derived class, then the derived method is called. This definition is weak; an example is better, as usual:
Almost a year ago now I promised a series of articles on cross-platform C++. Since then, I have finished porting a relatively large C++ project that I wrote to Linux, Solaris, and Windows. I’ve learned that C++ is really quite portable and there are just a few guidelines that you should keep in mind.
Jon asks:
GCC 4.4.0 was released on April 21st. Notable changes include fascinating new optimizations for loops. Particularly, they include the ability for the compiler to automatically rewrite loops to take into account memory layout of the system. I recall distinctly in my Intro to Data Structures CS class the teacher using the example of a two dimensional loop which iterated the “wrong way” (causing many disparate memory look ups and cache-misses) as a classic performance killer. Example (from GCC’s website), GCC is now able to perform the following optimization:
When declaring a template you can choose either “class” or “typename” for a template parameter. Example:
The first Project Euler problem is to calculate the sum of all integers below 1000 which are divisible by either 3 or 5. My solution is implemented entirely in C++ templates. The value is recursively calculated at compile time. The template specialization struct Problem1<0>
stops the recursion and returns 0. To compile this code with gcc you must expand the maximum allowed template recursion depth to at least 1000. ` g++ EulerProblem1.cpp -ftemplate-depth-1000`
If you search around for how to build Visual Studio projects from the command line you will find references to the DevEnv executable. When working with Visual C++ Express, however, that tool does not exist.
Most of the postings I make to this website are for my own personal reference. They are things that I want to make sure I don’t forget, or at least have easy access to. Today is no exception. For some reason, I can never remember the following command and have to track it down every time I’m interested.
The boost developers mailing list recently discussed the differences between reinterpret_cast<>
and static_cast<>
C++ casting operators.
Different compilers treat the two types of includes:
I recently had the need to be able to query the WMI via WQL (which are very fascinating and helpful technologies, actually) from C++. WQL essentially provides an SQL interface for querying system properties of a running computer.
The boost::lexical_cast<>
utility is a handy way of converting objects to and from strings, providing a mechanism that many scripting languages have built in. lexical_cast works with any C++ type that has ostream and istream operators defined for it, that is, any object that cout << object;
or cin >> object;
would work with. This boost facility also does intelligent things such as throwing a bad_cast exception if the operation causes an error flag on the stream to be set. I use boost::lexical_cast<>
throughout my code at work for things like serialization of objects for human readable communications. It is also sprinkled liberally throughout my code for handling things like quick debug logging:
Sometimes, in the course of C++ template based programming it might be desirable to have a constructor that is templated, like the following contrived exampled:
I’m sure I will get some dissenting views posted in comments regarding this, but I just came to this conclusion while trying to track down a memory bug in the past few days. First of all, what’s the difference? Take the follow code to set up our scenario:
Short answer: don’t do it! Long answer: As the C++ example from the article on Real World Haskell Chapter 1-2 showed:
I was recently faced with the following code which is simple but provided the perfect example to practice some Haskell.
Chapter 3 of Real World Haskell is “Defining Types, Streamlining Functions.”
Chapter 1 of Real World Haskell covers the most basic aspects of the language, such as common operators and operator precedence and gives some overviews of aspects of the language.
In case you find yourself asking where I get the stuff from that I blog about and wondering if I’m just making stuff up; many of the “Nobody Understands C++” articles on this website are inspired by principles found in the book “C++ Coding Standards” by Herb Sutter and Andrei Alexandrescu.
It is best to avoid using pointers in C++ as much as possible. The use of pointers can lead to confusion of ownership which can directly or indirectly lead to memory leaks. Even if object ownership is well managed simple (and difficult to find) bugs can also lead to memory leaks.
I do not, contrary to the opinions of some (rather weepy) individuals, ask interview questions like, “name the types of C++ inheritance” then flog the interviewee for saying “virtual, non-virtual, single, multiple,” because I was expecting “public, private, protected.” Indeed, it’s been more than two years since I last had the opportunity to interview, but when I did, I made sure of two things:
Release 0.0.2 of Swig Starter Kit was just released. This release sees the addition of template usage examples, including custom function templates and STL usage. Using a SWIG template declaration we are able to instantiate a specific template and use it from our script code.
I’ve heard this question come up a few times in C++ programming circles, “Why is ++i faster/better than i++?”
I felt like this topic deserved one more article. (See part 1 and part 2 for the background.)
After the first article on loop optimization my cousin pointed out to me that in some cases, tail recursion can actually be faster then loops in C with the help of tail recursion elimination.
I recently had a friend point out to me that your typical loop seen in every day code has minor inefficiencies in it which can add up to a good amount of time being wasted. I agreed with him, so I set out to write an article on how to optimize your C++ loops.
There is probably a multitude of ways that you can work too hard as a C++ developer, but there are two specific ones that I would like to focus on:
This is the first in a series of articles on writing crossplatform applications with C++. These articles will be based on the premise that strict adherence to standard C++ will result in extremely portable code which will run on almost every platform available, including embedded systems. However, limiting yourself to strict C++ also means limiting yourself to the standard C++ libraries, which only cover basic file and console input and output. That means no graphical user interfaces. This is certainly a limitation we will address. We will progressively add in more and diverse libraries which will slowly limit the full range of platforms we can run on.
Pass by iterator is not a new concept, but one that we are going to perhaps give a new name to today and propose as a standard for normal usage. If you take for example the normal way of passing around standard containers:
We’ve now completed 5 articles in the “Nobody Understands C++” series so here we are going to recap the misconceptions we have covered.
On occasion you will read or hear someone talking about C++ templates causing code bloat. I was thinking about it the other day and thought to myself, “self, if the code does exactly the same thing then the compiled code cannot really be any bigger, can it?” Here are the test cases presented, first without the use of templates, second with the use of templates. Exactly the same functionality and exactly the same code output:
Standard Algorithms Few C++ developers seem to appreciate that the standard C++ library is actually designed around functional programming principles.
We’ve covered the “Assembly Language”, “C” and “C++” of the C++ threading world, and now we are going to try and move beyond that.
I’m going to cover a thread safety strategy I have been thinking about lately. Let’s look at an example for a typical “lock the variables as you use them” approach:
In my last posting about C++ Multiple Dispatch I wondered if it was really any different than function overloading. I now appreciate that it is something that needs to occur at runtime, not compile time.
I clearly must be missing something. I just noticed this article on the O’Reilly ONLamp blog, discussing multiple dispatch in Perl. The example code given:
C++ templates is a huge topic that we will not fully cover here. While we have covered templates in the past, this article will cover the very basics and the reasons why we would want to use templates.
If boost::threads represent the C of multithreaded programming, then RAII and automatically managed threads represent the C++ of multithreaded programming. In the last article we promised that using more RAII would allow us to get this code even smaller and better to manage. Here is the result of that:
A while back I was going though the first chapters of Knuth’s The Art of Computer Programming and came across Euclid’s algorithm for finding the greatest common denominator. I decided to implement the algorithm as a C++ template. Here’s the complete example.
Note 2016-03-15 std::threads now have all this and more
Note that some of the details on volatile
are out of date now - 2016-03-15
Jon asks:
The follow code demonstrates a method for generating the Fibonacci Sequence at compile time. Since the sequence is generated at compile time runtime execution of retrieving a number is constant time.
This is going to be the first post of several, though I’m not sure how many just yet. The title of the post should be self explanatory, but I will elaborate. It is my strongly held belief that C++ is generally poorly understood and gets a bad rap because of this. It is also my belief that this is NOT the fault of C++, but rather the fault of old-school C++ developers who learned the language before the language was ratified in 1998.
Say you come up with a clever idea for initializing standard container types (blatantly stolen from the C++0x Initializer List concept). It might looks something like the implementation below:
The boost spirit library allows for direct translation of a BNF grammar into C++ code which generates a parser at compile time. The following example, from http://spirit.sourceforge.net/distrib/spirit_1_8_3/libs/spirit/doc/introduction.html truly does this concept more justice than I could: BNF Example:
Just a few days ago I spent way too long looking at the boost libraries to see if there was a way of adapting a std::fstream to an iterator type. I didn’t find what I was looking for and did what I wanted a completely different way.
boost contains a preprocessor metaprogramming libary. What this means, simply, is that it is possible to write code which generates code. The full docs are here.
Let’s say I’m doing some cryptography work. I’ve just received a signed message from another machine and I want to validate the signature. I have a few options:
Today I will be covering all 4 of the C++ cast operators.
In an effort to reduce the number of nonconstructive comments posted by visitors who do not read the entire article or previous comments, I have decided to change the title of the article and the initial summary to something more accurate.
Milestone 3.7 was released on Sourceforge today. This release adds the ability to build a Perl extension for using the Crate Game Engine library from within Perl.
Milestone 3.6 of the Crate Game Engine was release to sourceforge today. This release adds support for generating a Swig based wrapper extension to the library for use with Python.
Last night I proved the expat is going to work for me by getting game object models loading. Still missing a few pieces, like loading of actions and connections from the .xml file. I expect in the next day or few to have game loading fully working and be able to release a new version. This time I will also release a windows build to go with it.
What follows is a posting that I made to the wxMozilla-devel mailing list. I promised viewers of this site that I would post info on how to build biblestudy under Cygwin/MinGW, so here it is, please post comments if you have any questions.
Well, noone responded to my last blog, so I went ahead and decided to use the expat library. I’ve encapsulated the function of loading an xml file into a “WorldLoader” class. None of this is checked in, but in the next day or two I expect to be able to load a world from an xml file and maybe I can get some other people involved in making games for the fledgling engine.